If you are having trouble with your C# code and the exception ‘string was not recognized as a valid DateTime’, you can find more information about C# and the cause of this exception. We will also show you examples that yield this error and the correct syntax in this guide.
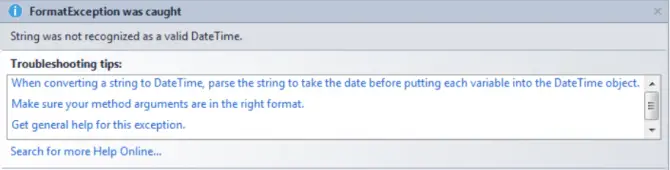
About C#
C# is a popular modern, object-oriented programming language. Microsoft first developed this language, pronounced C-sharp, in 2000. C# was released in conjunction with the .NET framework and Visual Studio. C# is part of the Common Language Infrastructure (CLI) (again developed by Microsoft), meaning it’s a language that can be run on many different computer systems without requiring the code to be changed.
Developers use the .NET framework, Microsoft’s primary software framework, to build and run applications for the Windows operating system.
Visual Studio is an incredibly popular and robust IDE used to write code, develop software, create websites, and more.
Why should you learn and use C#?
You are going to hit stumbling blocks and lots of error codes, like the one that brought you here, when learning a new language. It’s good to remind yourself why C# is in such demand and why you should continue to learn and hone your skills. Below are a few of the top reasons to learn C#:
1. High-Level Language
C# is a high-level language, meaning it’s more like the human language than machine code. This makes C# much easier to learn than C, C++, Perl, and assembly.
2. Game Development
C# was developed for Microsoft products, which dominate the operating system market. When game developers want to create new games, they often choose C#. For example, C# is used for game development in the Unity game engine.
3. Web and Desktop Applications
C# is also highly popular for developing web and desktop applications. When developers create applications that will run on Windows operating systems, they are likely to choose C#.
4. Large Community
With a Microsoft product comes a large community. Being a part of a large community of developers means you have access to mentors, help, and new tools and software that are constantly being developed.
What does the ‘string was not recognized as a valid DateTime’ error message mean?
The ‘string was not recognized as a valid DateTime’ exception is raised when you are incorrectly trying to transform a string into a DateTime object. In C#, the DateTime class combines both date and time into one object. DateTime is a struct type that contains the methods IComparable, IFormattable, IConvertible, ISerializable, IComparable<DateTime>, and IEquatable. Because DateTime is a Value type, it must have a value and cannot be assigned a null value.
This error appears most commonly when you try to parse a string to a DateTime object. You may attempt to parse a string to a DateTime object when you need to perform operations such as the WeekDay property, which will tell you the weekday of a specific date.
There are a few build-in methods that can be used to convert from string to DateTime object. Follow the links if you need help deciding which one to use or need further documentation:
- Convert.ToDateTime(string) – converts specified string into DateTime object
- DateTime.Parse(string) – culture-sensitive, parses specific string into DateTime object
- DateTime.ParseExact(s, format, provider) – culture-sensitive, parses a specified string into DateTime object, the format of the string must match specified format EXACTLY
- DateTime.TryParse(s, provider, styles, result) – converts a specified string to DateTime object and returns value to indicate if the conversion succeeded
- DateTime.TryParseExact(s, format, provider, style, result) – parses specific string into DateTime object, format of string must match specified format EXACTLY, returns value to indicate if the conversion succeeded
Some general examples for converting or parsing a string into DateTIme object are displayed below:
CultureInfo culture = new CultureInfo("en-US");
DateTime dateTimeObj = Convert.ToDateTime("11/11/2011 11:11:11 PM", culture);
DateTime dateTimeObj = DateTime.Parse("11/11/2011 11:11:11 PM");
DateTime dateTimeObj = DateTime.ParseExact("11-11-2011", "MM-dd-yyyy", provider); // 11/11/2011 11:00:00 AM
DateTime dateTimeObj; // 11-11-2011 11:00:00 AM
bool isSuccess = DateTime.TryParse("11-11-2011", out dateTimeObj); // True
DateTime dateTimeObj; // 11/11/2011 11:00:00 AM
CultureInfo provider = CultureInfo.InvariantCulture;
bool isSuccess = DateTime.TryParseExact("11-11-2011", "MM-dd-yyyy", provider, DateTimeStyles.None, out dateTimeObj); // True
What is CultureInfo?
If you notice, the first line in the code block above references culture and CultureInfo. CultureInfo is a class that contains a set of conventions that are assigned to a specific culture. The conventions that contribute to DateTime include:
- Number formats (commas or periods)
- Different date and time formats
- Calendar specifics for different countries
Why do you get the ‘string was not recognized as a valid DateTime’ error message?
1. String is not in DateTime Format
When parsing from string to DateTime, a variety of date and time formats are expected. If the string does not match any of the formats, then the ‘string was not recognized as a valid datetime’ exception will be raised:
DateTime dateTime10 = DateTime.Parse(dateString);
dateString = "this is not a date format";
// Exception: The string was not recognized as a valid DateTime.
// There is an unknown word starting at index 0.
In this example, the string ‘this is not a date format’ is assigned to the variable dateString. When the method DateTime.Parse() is applied to the object dateString, an exception is thrown because the content ‘this is not a date format’ is in no way a DateTime format.
2. Missing CultureInfo
Different cultures around the world use different order, calendars, and punctuation for their DateTime formats. If your data contains the strings in one DateTime format, but you are trying to parse it into another DateTime format, this can cause issues.
A date like 20/11/2011 may throw an exception when parsing if the culture is not specified, especially if it is trying to use the ShortDatePattern (MM/dd/yy) for the United States (en-US). An exception will be raised:
tempDate = “20/11/2011 11:11:11 PM”
DateTime tempDate = Convert.ToDateTime(“20/11/2011 11:11:11 PM”, en-US);
3. String does not match DateTime format
Often, you are trying to carry out operations with your data. If you are using DateTime.ParseExact() and are getting the ‘string was not recognized as a valid DateTime’, you should check the format you passed with your input.
For example:
DateTime.ParseExact(row("Date").ToString.Trim,"dd/MM/yyyy HH:mm:ss",System.Globalization.CultureInfo.InvariantCulture)=Today
The date in the “Date” row that you are attempting to parse is:
25/7/2020
15/3/2020
29/6/2020
The data is in the format dd/M/yyyy and you are trying to change it to dd/MM/yyyy HH:mm:ss. You need to make two changes to allow this to pass without exception.
How to parse a string into DateTime without the ‘string was not recognized as a valid DateTime’ error message in C#
If you need help correcting the syntax errors, like those in the examples listed above, the solutions are listed below:
1. String is not in DateTime Format
Again, the issue here is you are passing a string that has no DateTime information to be parsed into a DateTime object. You need to check that your string can be interpreted as a DateTime. Let’s recap the problem:
DateTime dateTime10 = DateTime.Parse(dateString);
dateString = "this is not a date format";
// Exception: The string was not recognized as a valid DateTime.
// There is an unknown word starting at index 0.
These are some DateTime formats that you can use:
- MM/dd/yyyy
- dddd, dd MMMM yyyy
- dddd, dd MMMM yyyy HH:mm:ss
- MM/dd/yyyy HH:mm
- MM/dd/yyyy h:mm tt
- MM/dd/yyyy HH:mm:ss
- MMMM dd
- ‘yyyy’-‘MM’-‘dd’T’HH’:’mm’:’ss.fffffffK
- ddd, dd MMM yyy HH’:’mm’:’ss ‘GMT
- yyyy’-‘MM’-‘dd’T’HH’:’mm’:’ss
- HH:mm
- hh:mm tt
- H:mm
- h:mm tt
- HH:mm:ss
- yyyy MMMM
- yyyy MMMM
If you have any questions about what the formats represent and what the result will be, you can get more information here.
The correct syntax for this example, you would need to reassign the input string to a valid DateTime format. Let’s use the ‘yyyy’-‘MM’-‘dd’T’HH’:’mm’:’ss.fffffffK format.
dateString = "2011-11-11’T’11:11:11.00.00’Z’";
DateTime dateTime10 = DateTime.Parse(dateString);
2. Missing CultureInfo
If you are missing the CultureInfo object, this exception can arise depending on the DateTime format requested. You need to include the CultureInfo object, use InvariableCulture (or CurrentCulture, depending on your inputs), or use ParseExact(). All three options are shown below:
1. ParseExact()
By using ParseExact(), no culture is needed because you are passing in the exact format string that is expected.
tempDate = “20/11/2011 11:11:11 PM”
DateTime tempDate = DateTime.ParseExact(tempDate, "dd/MM/yyyy");
2. CultureInfo
Let’s look at an example that displays the importance of the CultureInfo object by using Great Britain as a culture. Great Britain is an English-speaking country, that uses the international DateTime format, dd/MM/yy. A date like 20/11/2011 may run into parsing issues if the culture is not specified, especially if it is trying to use the ShortDatePattern (MM/dd/yy) for the United States (en-US). This may throw an exception:
tempDate = “20/11/2011 11:11:11 PM”
DateTime tempDate = Convert.ToDateTime(“20/11/2011 11:11:11 PM”);
Now, let’s change this to an applicable culture that can accept the format of this string. To specify the culture for Great Britain:
CultureInfo culture = new CultureInfo(“en-GB”);
DateTime tempDate = Convert.ToDateTime(“20/11/2011 11:11:11 PM”, culture);
3. CultureInfo.InvariantCulture
If you do not need to specify a culture for your needs, you can use the culture-independent object, InvariantCulture. InvariantCulture has no cultures assigned to it, although it is associated with the English language. Invariant Culture is best used when the string is not provided by the user:
DateTime tempDate = Convert.ToDateTime(“20/11/2011 11:11:11 PM”, CultureInfo.InvariantCulture);
If the user is supplying the string, then you should instead pass CultureInfo.CurrentCulture. This will grab the settings that the user has specified in the Regional Options in the Control Panel:
DateTime tempDate = Convert.ToDateTime(“20/11/2011 11:11:11 PM”, CultureInfo.CurrentCulture);
3. String does not match DateTime format
This example is similar to the first, but instead of having a string without any date or time information, you have a string but are trying to use the wrong DateTime format. Sometimes it is easier to change the format you seek for the DateTime object than to change the data. This will depend on the project and your needs. In this example, the DateTime format requested will be changed. Let’s recap the problem:
DateTime.ParseExact(row("Date").ToString.Trim,"dd/MM/yyyy HH:mm:ss",System.Globalization.CultureInfo.InvariantCulture)=Today
The date in the “Date” row that you are attempting to parse is:
25/7/2020
15/3/2020
29/6/2020
The data is in the format dd/M/yyyy and you are trying to change it to dd/MM/yyyy HH:mm:ss. You need to remove the MM format and adjust it to M. MM requires two numbers, but M can work with one or two numbers. You also need to remove the HH:mm:ss formatting, as there is no specific time information within the data:
DateTime.ParseExact(row("Date").ToString.Trim,"dd/M/yyyy",System.Globalization.CultureInfo.InvariantCulture)=Today
Fixed: ‘string was not recognized as a valid DateTime’
Learning about the DateTime object and how to properly use its methods is important to avoid the ‘string was not recognized as a valid DateTime’ exception. The methods are used in different situations and allow different parameters to be passed in. Understanding the different DateTime formats available and the CultureInfo class are also significant in selecting the correct format for your data. If you followed the examples and syntax corrections above, you should have successfully cleared the exception.
If you are still having issues, you can find more specifics and examples in Microsoft’s Documentation for DateTime and CultureInfo.