What is the err_unknown_url_scheme Android WebView error?
The err_unknown_url_scheme error usually comes up during Android WebView app development when a developer has chosen links to have mailto://, whatsapp://, or any other intent that’s not the basic http:// or https://. Specific URL coding needs to be added to handle these difference URL schemes to prevent this error.
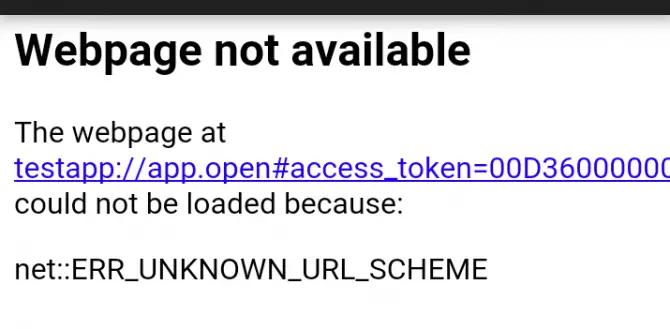
The discussion and solutions for this article are directed towards Android developers. If you are a user and run into this error, there’s nothing you can do to immediately solve the problem. Your best course of action would be to contact the app developer to make sure they are aware of this bug.
Android Dominance
Android is the dominant operating system (OS) for mobile devices. Android boasts a 71% market share, followed by Apple’s iOS with 27% market share. Google bought Android Inc. in 2005 in order to have control over Android’s future development and to load Android OS on all of its mobile devices. All other major mobile device manufacturers, besides Apple, use Android. Samsung, OnePlus, Huawei, Motorola, Nokia, LG, and Sony devices all come preloaded with the Android OS. It’s also significantly easier to develop and host an app on Google’s Play Store than on Apple’s App Store.
These factors have contributed to a robust Android developer community. Android developers use meetups and conferences, slack and Reddit, blogs and forums, and Stack Overflow to communicate and learn from each other. Many developers are self-taught and rely on the insights of the Android community to overcome persistent programming errors and bugs.
Android Studio is the only official development environment for Android. Android Studio was built using JetBrains’ software, the same company responsible for a variety of other popular IDEs (integrated development environments) for languages like Python (Pycharm), PHP (PhpStorm), and Java (IntelliJ IDEA).

What are Native Apps? How are they related to the err_unknown_url_scheme error?
Native apps live in the Google Play Store or App Store. They are fully developed applications that are installed directly onto the mobile device. Native apps will maintain most of their functionality even when not connected to the internet or mobile networks. These differ from web apps, which are simply websites. Web applications are easier to develop but don’t have access to any system features like GPS.
As a developer you may want to build a native app but still provide access to web content. This is done using WebView. WebView is a special class that allows web content to be displayed within your native app. For example, if you have the native Facebook app on your phone and click an external link, the webpage will load within the app. You can navigate around on the loaded webpage, access other parts of the webpage, shop, and interact. The embedded browser will not have an address bar for your user to search with. This provides the developer a way to have both the benefits of a web app and a native app.
WebView is set to handle certain URL schemes. If you try to use a special URL scheme outside the standard https:// and http://, WebView can throw the err_unknown_url_scheme error. A few of the non-standard URL schemes that WebView isn’t set to recognize are:
- mailto://
- whatsup://
- file://
- telnet://
- intent://
- market://
- app://
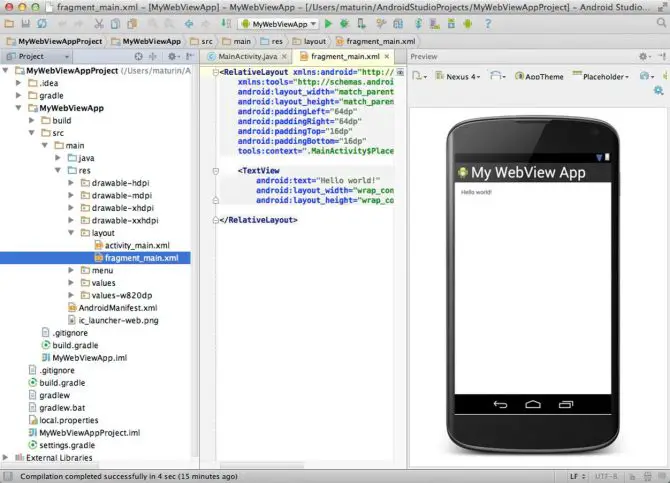
3 ways to fix the err_unknown_url_scheme error
To solve this error, you can go one of two routes: either disable those schemes and only develop your app using http:// and https:// schemes, or you can edit your code to be able to handle these specific URL schemes. The first option is not an absolute solution but can fix the problem in some use cases. The second solution shows examples of how to add new intents for URL schemes, and the third solution involves disabling any non-standard URL schemes.
1. Open In New Window
A quick and easy stopgap solution is editing your URL href code. By adding target=”_blank” you are able to solve the problem in a roundabout way. Now adding this snippet of code can have an undesirable development effect because this link will now open in a new window.
Development best practice is to avoid opening extra browser windows. This is because a new browser can bother or confuse your user. By removing the ‘back’ button, users find it more difficult to navigate and get back to their previous page in your app. The standard is target=”_self”, which opens the link in the same browser page.
Below is an example of URL href code with target=”_blank” added in:
<a href= “tel:555-111-5555” target=”_blank”> Contact Me </a>
Or:
<a href=”mailto:myname@gmail.com” target=”_blank”> Email Me </a>
2. Add New Intent to Load in External App
In Android development, intents are used to tell Android what you want the app to do, essentially allowing you to call a specific action or component. Some scheme errors can be fixed by adding an intent to load in an external app. For example, loading mailto:// in an external app should cause the email link to open in your default email application. Another example is maps:// which should open in your default maps application.
In the code snippet below, you will be using an IF statement to handle this. Any URL that starts with http:// or https:// is ignored and opened as usual. Otherwise, if the URL involves a special scheme such as:
- tel://
- sms://
- mailto://
- geo://
- maps://
then a new intent is added to load the action in an external app. If the activity cannot be successfully started because no associated external app exists on that system, an error will appear to the user.
@Override
public boolean shouldOverrideUrlLoading(WebView view, String url) {
if (url == null || url.startsWith("http://") || url.startsWith("https://")) {
return false;
}
try {
Intent intent = new Intent(Intent.ACTION_VIEW, Uri.parse(url));
view.getContext().startActivity(intent);
return true;
} catch (Exception e) {
Log.i(TAG, "shouldOverrideUrlLoading Exception:" + e);
return true;
}
}
Another code snippet is included below to show that you can tackle this problem in a variety of ways. This method overrides URL loading like seen above and should open a related app to carry out the protocol. If the associated application, in this case Whatsapp is not installed, a message is displayed for your user. This message is called a toast message and gives the user an idea on what went wrong and how to fix it. The toast message for this scheme was ‘Whatsapp has not been installed’.
@Override
public boolean shouldOverrideUrlLoading(WebView view, String url) {
view.loadUrl(url);
if (url.startsWith("whatsapp://")) {
webview.stopLoading();
try {
Intent whatsappIntent = new Intent(Intent.ACTION_SEND);
whatsappIntent.setType("text/plain");
whatsappIntent.setPackage("com.whatsapp");
whatsappIntent.putExtra(Intent.EXTRA_TEXT, webview.getUrl() + " - Shared from webview ");
startActivity(whatsappIntent);
} catch (android.content.ActivityNotFoundException ex) {
String MakeShortText = "Whatsapp has not been installed";
Toast.makeText(WebactivityTab1.this, MakeShortText, Toast.LENGTH_SHORT).show();
}
};
};
3. Disable any non-standard URL schemes
By disabling URL schemes other than the standard https:// and http:// you are bypassing this problem completely. You won’t be able to use any custom schemes like the ones listed earlier in the article.
If your app has one of these schemes or a user somehow navigates to a link using these schemes, a toast message will appear. The message “Error: Unknown link type” will tell the user they can’t proceed. Below is an example of this method:
@Override
public boolean shouldOverrideUrlLoading(WebView view, String url) {
view.loadUrl(url);
if (url.startsWith("http") || url.startsWith("https")) {
return true;
}else {
webview.stopLoading();
webview.goBack();
Toast.makeText(MainActivity.this, "Error: Unknown link type", Toast.LENGTH_SHORT).show();
}
return false;
}
WebView Has Great Benefits, But Some Creative Coding Is Needed
Android WebView really provides the best of both worlds for Android developers, but creative workarounds are needed for some situations. By using WebView to display an embedded browser within your native app, you gain all the benefits of both the native app and a web app. You will be able to use the native system APIs in your web code which is usually constrained.
If you are developing an app and want to include special URLs, outside of http:// and https:// you will need to add some intents or fall back URLs, otherwise your our users will experience the error “err_unknown_url_scheme”. When a URL like mailto:// or maps:// is called, your code needs to open an associated external app that can complete that action. Otherwise you can disable these other URLs and set a specific error message to any users that stumbles upon a link with one of these URL schemes.
Net.err_unknown_url_scheme
Thank you so much
I’m having the err unknown problem. Can I get help to fix ?
thank you so much this work.